A Comprehensive Guide to IGCSE Computer Science Programming
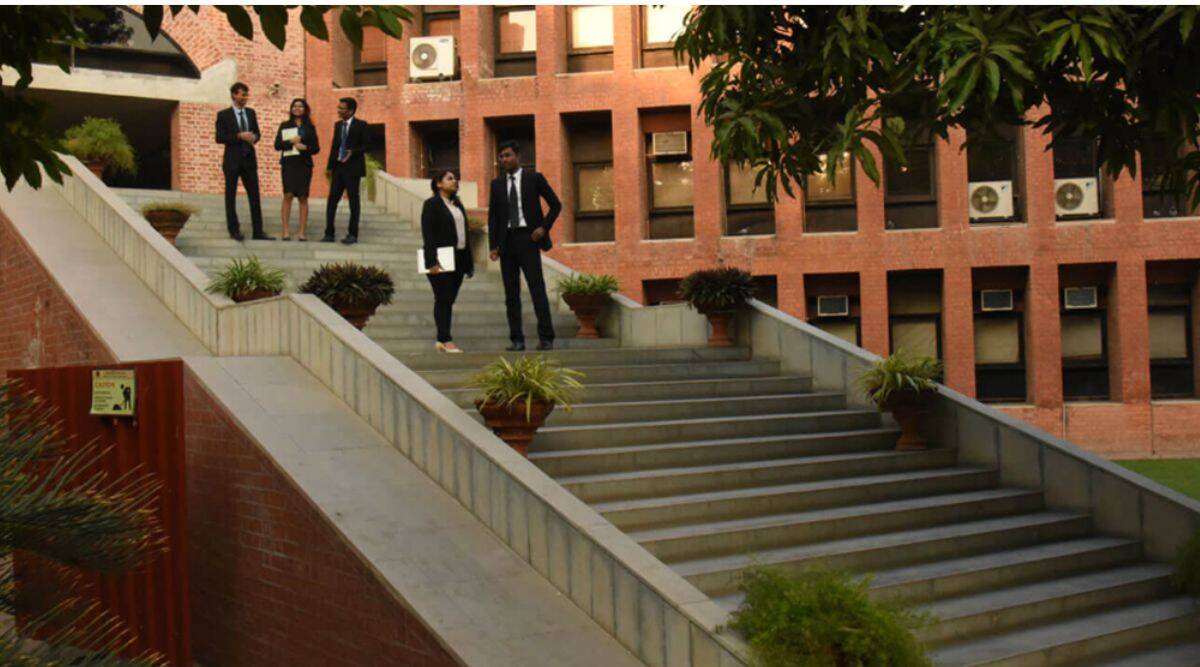
IGCSE Computer Science programming introduces students to the fundamental concepts of computer programming, equipping them with the skills to write, debug, and analyze programs effectively. In this comprehensive guide, we will explore the key principles, techniques, and strategies for mastering programming in IGCSE Computer Science. From understanding algorithms and data structures to implementing solutions and debugging code, this guide will provide students with the knowledge and tools to excel in programming tasks and assessments.
1. Understanding Programming Fundamentals:
Begin by understanding the fundamental concepts of programming, including variables, data types, operators, control structures, and functions. Familiarize yourself with programming languages commonly used in IGCSE Computer Science, such as Python, Java, or Scratch. Learn how to write clear, concise, and well-structured code that follows best practices and conventions.
2. Mastering Algorithms and Problem-Solving Techniques:
Develop proficiency in designing and implementing algorithms to solve a variety of computational problems. Learn how to analyze problem requirements, identify inputs and outputs, and devise step-by-step solutions using pseudocode or flowcharts. Practice breaking down complex problems into smaller, manageable tasks and implementing algorithms using programming constructs such as loops, conditionals, and functions.
3. Implementing Data Structures:
Explore different data structures, such as arrays, lists, stacks, queues, and trees, and understand their properties, operations, and applications. Learn how to choose the appropriate data structure for different problem scenarios and implement them using programming languages' built-in data structure libraries or by defining custom data structures. Practice manipulating data structures, performing operations such as insertion, deletion, searching, and sorting efficiently.
4. Debugging and Testing Techniques:
Develop effective debugging and testing techniques to identify and correct errors in your code. Learn how to use debugging tools and techniques to trace program execution, identify logical errors, and fix syntax errors. Practice writing test cases to validate the correctness and robustness of your programs and ensure they meet specified requirements. Learn how to interpret error messages and use them to diagnose and troubleshoot issues effectively.
5. Applying Object-Oriented Programming Principles:
Explore object-oriented programming (OOP) principles, including encapsulation, inheritance, polymorphism, and abstraction. Learn how to design and implement classes, objects, and methods to model real-world entities and relationships. Practice designing class hierarchies, implementing inheritance relationships, and using interfaces and abstract classes to achieve modularity, extensibility, and code reuse.
6. Developing Graphical User Interfaces (GUIs):
Learn how to design and develop graphical user interfaces (GUIs) for interactive applications using programming frameworks or libraries. Explore GUI design principles, layout managers, event handling, and user input validation techniques. Practice creating GUI components such as buttons, labels, text fields, and menus, and wiring them together to create functional and user-friendly interfaces.
7. Integrating File Handling and Input/Output Operations:
Understand how to perform file handling and input/output operations in your programs to read and write data from external files or devices. Learn how to open, read, write, and close files using file streams or input/output streams. Practice handling exceptions and errors that may occur during file operations and implementing error handling mechanisms to ensure robustness and reliability.
8. Optimizing Performance and Efficiency:
Explore techniques for optimizing the performance and efficiency of your programs, such as algorithm optimization, code refactoring, and memory management. Learn how to analyze program performance using profiling tools and techniques and identify bottlenecks and areas for improvement. Practice implementing optimization strategies such as algorithmic improvements, data structure optimizations, and code optimizations to enhance program speed, scalability, and resource utilization.
Conclusion:
Mastering programming in IGCSE Computer Science requires a solid understanding of programming fundamentals, algorithms, data structures, debugging techniques, object-oriented programming principles, GUI development, file handling, and optimization strategies. By following the comprehensive guide outlined above and practicing regularly, students can develop the knowledge, skills, and confidence to excel in programming tasks and assessments. Whether pursuing further studies in computer science or entering the workforce, proficiency in programming is a valuable asset that opens doors to a wide range of opportunities in the digital age.